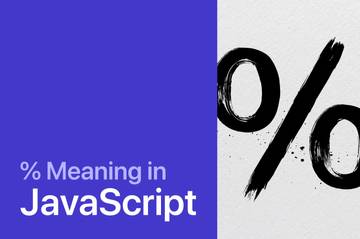
What Does % Mean in JavaScript?
JavaScript is a great language to learn for anybody interested in the tech industry. Almost 97% of the websites you see online use JavaScript, so it's only natural that many people are learning this skill. With so many syntaxes to learn, it can often get confusing. For instance, the % operator is something beginners have a hard time with. So, what does % mean in JavaScript?
The % sign or the modulus sign is an operator in JavaScript that is used to find the remainder of any two numbers. For instance, if you divide five by two, you would get one as the remainder:
5 % 2 // result is 1
This is because 2 is an inexact division, the division is 2.5, so 5 is 2 times 2 plus 1(remainder).
That is what the % operator is for. This operator is perfect to be used in loops and conditions to check even or odd numbers and many other calculations.
There are many other operators like the modulus in JavaScript, and they're all very useful for writing algorithms. Let's take a deeper look into these operators so you can have a better grasp of them. So, without further ado, let's get right to it.
What is JavaScript Operator?
As the name suggests, an operator can perform a specific operation on one, two, or multiple values or operands. It provides a result. You can think of them as a way to do calculations, even though operators are not only limited to arithmetic.
All programming and scripting languages have operators, and they usually work the same way. They are very useful and allow you to write conditions and functions and even perform calculations. Whether you're working on the backend or in the frontend, operators are going to appear again and again in your code.
Something as simple as declaring a variable requires an assignment operator (=). So it's safe to say operators are a fundamental part of any programming or scripting language. Operators have the power to manipulate data values and produce relevant results.
How Many JavaScript Operators are There?
There are mainly seven different types of JavaScript operators. These include arithmetic operators, logical operators, comparison operators, assignment operators, string operators, bitwise operators, and miscellaneous operators. Each of these overall groups has multiple different operators that you can use for many functions.
Let's check out what each of these does.
Arithmetic Operators
As the name suggests, arithmetic operators are used for regular arithmetic calculations. There are eight different arithmetic operators, and these are addition (+), subtraction (-), multiplication (*), division (/), modulus or remainder (%), increment (++), decrement(--) and exponentiation or to the power of (**).
All of these can be used in different calculations and conditions. They are very handy, as most systems do require small calculations here and there.
Logical Operators
Logical operators usually come in very handy in conditions and writing functions. They perform logical operations and return a boolean(true or false) value. JavaScript has three different logical operators, and these are AND (&&), OR (||), and NOT(!).
The AND operator checks if all conditions mentioned are true, the OR condition checks if any of the conditions mentioned are true, and the NOT condition is used to check if a condition is not true.
If you've learned how to write algorithms, you'll know how important logic is in any programming or writing code. These are used for making your code take decisions.
Comparison Operators
Comparison operators are also used in writing conditions and loops. They compare two values and return a Boolean value of whether the condition is true or false. For example, the expression:
console.log(2>3)
would print false because "2" is not greater than "3".
Expressions involving comparison operators are often used with logical operators or assignment operators to write functions. There are "8" different comparison operators, and these are:
- Equal to (==)
- Not equal to (!=)
- Strict equal to (===)
- Strict not equal to (!==)
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=).
Assignment Operators
These are used to assign or provide a value to different variables. For example, if you want to create an age variable and store a digit value in that variable, you need to use an assignment operator.
Strictly speaking, there is only a single type of assignment operator, and it is "=". However, the = symbol is often used with other arithmetic symbols to perform more complex assignments. For instance, -= is a subtraction assignment. It will subtract "1" from the operand before assigning the value to the variable.
String Operators
You can use the addition operator, the + sign to concatenate or join two separate strings into one whole one. It's like adding two sentences together. So the addition operator can also be defined as the string operator when used with strings.
Depending on what the operand is, the meaning of this operator changes. It is very handy when it comes to adding different string variables.
Bitwise Operators
These are a kind of operator that is barely used in frontend work or regular programming. These are used to perform bitwise operations when using binary representations of numbers. If you've studied JavaScript in school, then you've probably had to use these in performing bitwise calculations.
There are "7" bitwise operators, and these are:
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise NOT (~)
- Left shift (<<)
- right shift (>>)
- Zero-fill right shift (>>>)
You might never use them, but it's always good to know that they exist.
Others
Then the other miscellaneous operators do not fall under any of the above categories. These things like the "delete" operator, which can delete an object's property, or the "void" operator, which allows evaluating expressions.
You'll find these out as you work more and more with JavaScript. The more you practice, the more you'll learn about the details of operators and how differently you can use each type.
How Does the % Operator Work in JavaScript?
Out of all the arithmetic operators, the modulus operator or % can be confusing for beginners because the other operators like addition or subtraction are things that most people have used in their daily lives. The % operator gives you the remainder of a division between two values.
We don't have a separate symbol in regular arithmetic to find the remainder, and we don't have a lot of use for it. But in coding, the % can be beneficial. For example, the most common use of the % operator is to check whether a number is even or odd.
Any even number will be divisible by "2" and give a remainder of 0. So to check if a number is even, you can find its modulus with 2. If the result is anything other than zero, then the number is odd; otherwise, it's even. These can be very useful in conditional functions.
Conclusion
I hope this article cleared any confusion you might have had about what does % means in JavaScript. The modulus operator, along with plenty of other operators, is an integral part of any programming language, and they have hundreds of uses. So it's always a good idea to keep on top of these functions and know how to use them.
If you have more confusion about other topics in the programming world or are just interested in frontend technology in general, then check out our other posts, and you'll find something that helps you out! We sincerely hope that this article was able to help you have a better understanding of JavaScripting. Thanks for tuning in.